Why Flutter rules in a nutshell
1) Flutter stinks because it uses a different language (Dart) and I don’t want to learn a new language

Dartis quite similar to C# in several aspects. In fact, it is more like a C# minus all the mumbo jumbo of C#.
For example, you don’t need “public”, “private”, “protected”, etc. Dart doesn’t have it (it has a prefix “_” for private but you are free to use it or not). In general, in Dart, every field is private, you can use setter and getters but it is not the way of Dart.
Second, the management of NULL is top notch and it is better than C#
In C# you could have: SomeClass instance=null;
In Dart, you could not have that variable that stores a null, no matter if it is an object or not. So, we will never ever have null pointers unless we explicit allow it. Dart is what we could call, a safe language.
But in general, if you know C#, then you know Dart.
In short, you can learn Dartin a weekend. The only “weirdness” is Stream and the constructors.
2) I don’t want to learn a new way to write a template

Flutter uses a different approach. It doesn’t use a template language at all. Everything is code. It is a nightmare if you want to use a fancy GUI editor but since practically every GUI editor is horrible (excluding XCode and IOS), then it is not a big deal. JSX, XAML, Blazor etc. are horrible (especially XAML), so you are free from them. Why they are horrible? It is because they are many differences between in what we draw versus in what we finally saw. For example, it is not the same to see an empty list versus a list full of data.

The advantage is the integration between the code and the “template” is seamless, because everything is code. If you learn Dart, you learn “templating Flutter”.
Plus: Flutter was designed and created thinking in Hot Reload, so it is easy to design a widget and see the changes instantly.

3) I don’t want to deal with fluid components
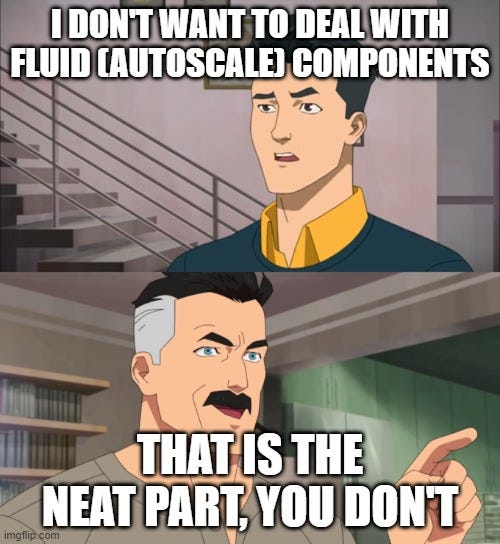
Most flutter Widgets don’t scale visually, and the few ones that scale, mostly use the whole space. You must do it on your own. This means, that we don’t deal with “anchors” or some wacky components that automatically screw our design.
4) And what about State management.

Every widget in flutter has their own fields but you don’t even need fields inside a widget to keep and draw some values.
Flutter works mainly with a simple function: setState() and nothing else much (the other is initState())
Flutter doesn’t work with state directly but with differences in the widgets. If the widget is considered “dirt”, then it draws only the parts that have changed, no matter the states or values. You can even use global variables as “states” or a function. Flutter doesn’t care, it simply works!.
5) I don’t want to learn a new framework

Flutter consists mainly in two kind of classes.
- Stateless Widgets
- Stateful widgets.
And nothing more!.
While Flutter has “states” but they are not the same as other frameworks. The states are just variables (field, global, a function, you name it). So you won’t find a complex flow to manage states such as Redux.
React Redux flow: (it is just one of many flows of react)

It is the flow of a Stateful widget (the green step are a simple function).
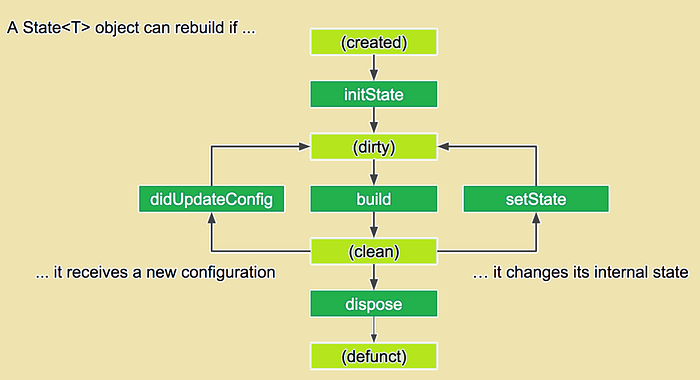
And Stateless widgets are the same, minus the setState() and didUpdateConfig().
Developing for Flutter is the same as to develop a pyramid

Why? We usually create code inside code, inside code inside code. We could split it creating widgets but finally everything is inside other thing (excluding the main function).